PUT/DELETE 메소드 사용 방법
방법 | 중요성 | CRUD | 멱등성 | 안정화 | 길 바꾸다 |
묻다 범위 |
데이터 본문 |
얻다 | 자원 획득 | R(읽기) | 영형 | 영형 | 영형 | 영형 | 엑스 |
우편 엽서 | 리소스 생성 및 추가 | C(만들기) | 엑스 | 엑스 | 영형 | △ | 영형 |
놓다 | 리소스 업데이트 및 만들기 | C/U(업데이트) | 영형 | 엑스 | 영형 | △ | 영형 |
삭제 | 리소스 삭제 | 남성 | 영형 | 엑스 | 영형 | 영형 | – |
머리 | 헤더 데이터 수집 | – | 영형 | 영형 | – | – | – |
옵션 | 지원을 받는 방법 | – | 영형 | – | – | – | – |
테슬라 | 반환 요청 메시지 | – | 영형 | – | – | – | – |
연결하다 | 프록시 작업을 위해 터널 연결로 변경 | – | 엑스 | – | – | – | – |
하나. PUT 업데이트(업데이트)
– 컨트롤러: PostApiController
@요청 본문 수정할 내용을 본문으로 받습니다. @경로 변수 수정을 위한 고유 ID를 받을 수 있습니다.
package com.example.put;
import com.example.put.dto.PostRequestDto;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class putApiController {
@PutMapping("/put/{userId}")
public PostRequestDto put(@RequestBody PostRequestDto postRequestDto, @PathVariable(name = "userId") Long id){
System.out.println(id);
System.out.println(postRequestDto);
return postRequestDto;
}
}
– 클래스(객체): PostRequestDto
@JsonProperty 위에서 설명한 json에서 사용하는 객체 이름을 선언하는 방법 외에,
전체 클래스 선언의 모든 이름을 무언가로 변경하여 확인하는 기능이 있습니다.
@JsonNaming(값 = PropertyNamingStrategy.SnakeCaseStrategy.class)
받은 이름을 스네이크 케이스 클래스로 변경합니다.
package com.example.put.dto;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.PropertyNamingStrategy;
import com.fasterxml.jackson.databind.annotation.JsonNaming;
import java.util.List;
@JsonNaming(value = PropertyNamingStrategy.SnakeCaseStrategy.class)
public class PostRequestDto {
private String name;
private int age;
private List<CarDto> carList;
@Override
public String toString() {
return "PostRequestDto{" +
"name="" + name + "\'' +
", age=" + age +
", carList=" + carList +
'}';
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public List<CarDto> getCarList() {
return carList;
}
public void setCarList(List<CarDto> carList) {
this.carList = carList;
}
}
– 클래스(객체): carDto
package com.example.put.dto;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.PropertyNamingStrategy;
import com.fasterxml.jackson.databind.annotation.JsonNaming;
public class CarDto {
private String carName;
@JsonProperty("car_number")
private int carNumber;
public String getCarName() {
return carName;
}
@Override
public String toString() {
return "CarDto{" +
"carName="" + carName + "\'' +
", carNumber=" + carNumber +
'}';
}
public void setCarName(String carName) {
this.carName = carName;
}
public int getCarNumber() {
return carNumber;
}
public void setCarNumber(int carNumber) {
this.carNumber = carNumber;
}
}
-결과
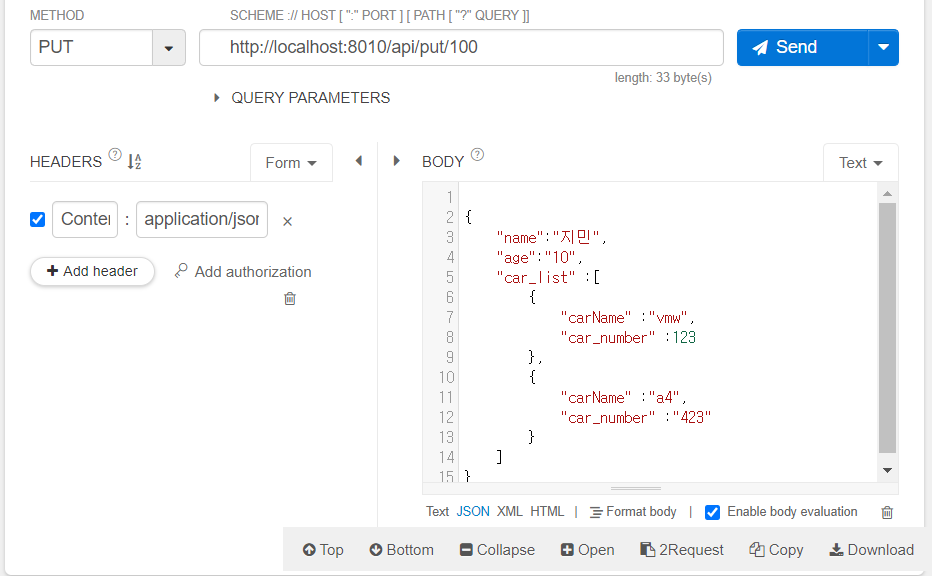
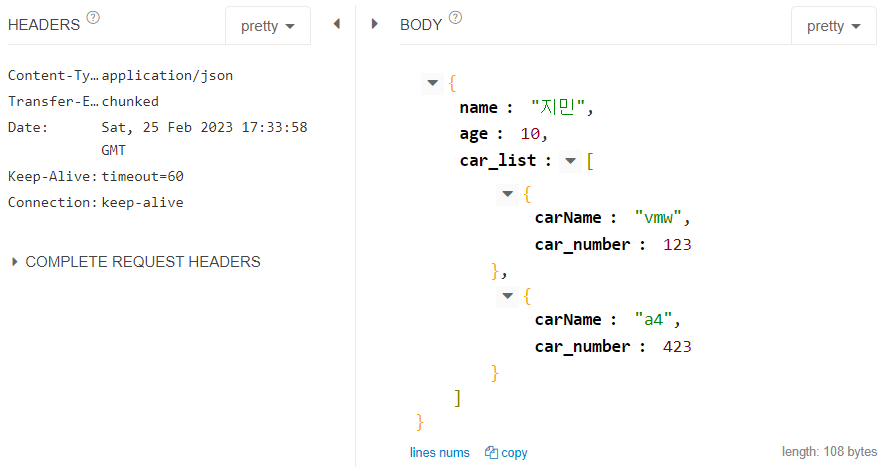
2. 삭제 삭제
http://localhost:8010/api/delete/{사용자 ID}?키=값
http://localhost:8010/api/delete/100?account=user100
@DeleteMapping 리소스 설정
삭제 기능의 경우 보통 고유번호를 직접 받아서 사용하거나 파라미터로 받아서 삭제하는 경우가 많습니다.
번호를 얻다 @경로 변수통과, ID 값은 @request 매개변수 로 받아들여
– 컨트롤러: DeleteController
package com.example.delete.controller;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class DeleteController {
@DeleteMapping("/delete/{userId}")
public void delete(@PathVariable String userId, @RequestParam String account){
System.out.println(userId);
System.out.println(account);
//delete 리소스 삭제, 제거할 값이 이미 없어도 동일하게 200 ok를 반환한다.
}
}
– 결과